SQL Coding
Improving Investigation & Information Warehousing With SQL
At Codelabpro, we make coding easier and enable learners with top-notch programming tutorials, courses, and resources. Whether you are a novice making your initial strides in coding or a seasoned developer aiming to improve your skills, we offer practical, real-world insights to assist you in achieving success.
SQL (Structured Query Language)
SQL (Structured Query Language) is a standardized programming language used for managing and manipulating relational databases. It allows users to perform various operations on the data stored in databases, such as querying, updating, deleting, and creating new databases, tables, and structures.
Key Features
- Data Querying: Retrieve specific data from large datasets using SELECT.
- Data Manipulation: Insert, update, and delete data using INSERT, UPDATE, and DELETE.
- Data Definition: Create and modify database structures using CREATE, ALTER, and DROP.
- Data Control: Manage permissions with GRANT and REVOKE.
- Transaction Control: Maintain data integrity with COMMIT, ROLLBACK, and SAVEPOINT.
Explanation
- SELECT: Specifies the columns to retrieve (e.g., name and age).
- FROM: Indicates the table to query (employees).
- WHERE: Filters rows where the condition (age > 30) is true.
SELECT name, age
FROM employees
WHERE age > 30;
Explanation of Core Concepts
1. Database Structure

2. Data Querying
SQL queries retrieve data from tables.
Explanation
- * retrieves all columns.
- The output would display every row in the employees table.
SELECT * FROM employees;
3. Data Manipulation
Modify data in the database using INSERT
, UPDATE
, and DELETE
.
Explanation:
INSERT
adds new rows to the table.UPDATE
modifies existing records.DELETE
removes rows based on conditions.
INSERT INTO employees (id, name, age, position)
VALUES (4, ‘Malik’, 28, ‘bester’);
UPDATE employees
SET age = 29
WHERE name = ‘Malik’;
DELETE FROM employees
WHERE name = ‘Malik’;
4. Joins


Example: Inner Join
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, luctus nec ullamcorper mattis, pulvinar dapibus leo.
SELECT employees.name, departments.dept_name
FROM employees
INNER JOIN departments ON employees.dept_id = departments.dept_id;
Result
INNER JOIN combines rows from both tables where the dept_id matches
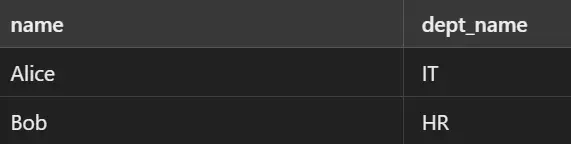
5. Aggregations
- COUNT counts the number of rows.
- AVG calculates the average value in a column.
SELECT COUNT(*) AS total_employees
FROM employees;
SELECT AVG(age) AS average_age
FROM employees;
6. Creating Tables
Explanation
- CREATE TABLE defines a new table.
- VARCHAR(50) specifies a text column with a maximum length of 50 characters.
- PRIMARY KEY uniquely identifies each row
CREATE TABLE employees (
id INT PRIMARY KEY,
name VARCHAR(50),
age INT,
position VARCHAR(50)
);
7. Constraints
Common Constraints:
ALTER TABLE employees
ADD CONSTRAINT age_check CHECK (age > 28);
8. Transactions
Explanation:
- BEGIN TRANSACTION starts a transaction.
- COMMIT saves changes to the database.
- ROLLBACK undoes changes.
BEGIN TRANSACTION;
UPDATE employees SET age = 26 WHERE name = ‘Alice’;
DELETE FROM employees WHERE name = ‘Bob’;
COMMIT; — Save changes
— ROLLBACK; — Undo changes (if needed)
Use Cases
- Data Retrieval: Reporting and analysis.
- Data Manipulation: Modifying data for business logic.
- Database Design: Structuring data with normalization.
- Data Integration: Linking multiple systems via databases.